Table Of Content

All the code examples in the book can be found in these pages. This repository focuses on the 23 famous GoF (Gang of Four) Design Patterns implemented in Python. Jacob is a software engineer with over 2 decades of experience in the field. His experience ranges from working in fortune 500 retailers, to software startups as diverse as the the medical or gaming industries.
Languages
It is time to complete the implementation of Factory Method and provide the creator. The creator in the example is the variable factory in ObjectSerializer.serialize(). The Song class implements the Serializable interface by providing a .serialize(serializer) method. In the method, the Song class uses the serializer object to write its own information without any knowledge of the format. The application can allow the user to select an option that identifies the concrete algorithm.
Gang of Four: Behavioral Patterns¶
Andrei is an IT professional with experience ranging from low-level programming to complex systems design and implementation. Despite the obvious utility in practice, design patterns are also useful for learning. They introduce you to many problems that you may not have considered and allow you to think about scenarios that you may not have had hands-on experience with in-depth. Patterns that could be applied to these sorts of problems are what we can meaningfully dub design patterns. The complete code for the reverse number pyramid pattern is given below. The left downward triangle pattern is the star pattern of a triangle upside down.
The SOLID Design Principles
Python, being a versatile and flexible programming language, allows developers to implement various design patterns. By using design patterns, you can separate concerns, improve code organization, and make your code more resilient to changes. Whether you’re working on a small project or a complex enterprise application, understanding and applying design patterns can greatly enhance your coding skills. Through the course of this article, we observed nine critical behavioral design patterns, each catering to specific challenges and scenarios commonly encountered in software design. The Factory Method pattern provides an interface for creating objects but allows subclasses to decide which class to instantiate. It promotes loose coupling and supports the “open-closed” principle, where new types of objects can be introduced without modifying existing code.
Naturally, the subclasses have the same fundamental properties, with some of their own particular ones. The OOP paradigm commonly leverages the usage of abstract classes, which are not a built-in feature in Python. To achieve this functionality, we use the ABC (Abstract Base Classes) library. A Design Pattern is a description or template that can be repeatedly applied to a commonly recurring problem in software design.
Low-level classes are the simple workers that perform actions, while high-level classes are the management class that orchestrate the low-level classes. Till now, our interface has exactly implemented the expected features. The implementation structure of ObjectFactory is the same you saw in SerializerFactory. Not all situations allow us to use a default .__init__() to create and initialize the objects.
Elegant RESTful Client in Python for Exposing Remote Resources - Packt Hub
Elegant RESTful Client in Python for Exposing Remote Resources.
Posted: Wed, 12 Aug 2015 07:00:00 GMT [source]
Object-Oriented Design in Python: The SOLID Principles
The initialization is a lot more clean and readable compared to the messy constructor from before and we have the flexibility of adding the modules we want. Notice that we've omitted specific initializations in the constructor, and used default values instead. This is because we'll use the Builder classes to initialize these values. Although they're different, they're somehow grouped together by a certain trait. However, the client decided to expand their business and will now provide their services to unemployed people as well, but with different procedures and conditions. Think of an event-driven system, like a GUI toolkit, where different UI components trigger specific actions when interacted with.
Technology Services
Lets you split a large class or a set of closely related classes into two separate hierarchies—abstraction and implementation—which can be developed independently of each other. Lets you produce families of related objects without specifying their concrete classes. The hollow alphabet pyramid pattern is a pyramid pattern that is made of alphabets and is hollow inside. The reverse alphabet pyramid pattern is a pyramid pattern that is made of alphabets and is upside down. The alphabet pyramid pattern is a pyramid pattern that is made of alphabets.
Finally, the application implements the concept of a local music service where the music collection is stored locally. The service requires that the the location of the music collection in the local system be specified. Creating a new service instance is done very quickly, so a new instance can be created every time the user wants to access the music collection. The current implementation of .get_serializer() is the same you used in the original example. The method evaluates the value of format and decides the concrete implementation to create and return. It is a relatively simple solution that allows us to verify the functionality of all the Factory Method components.
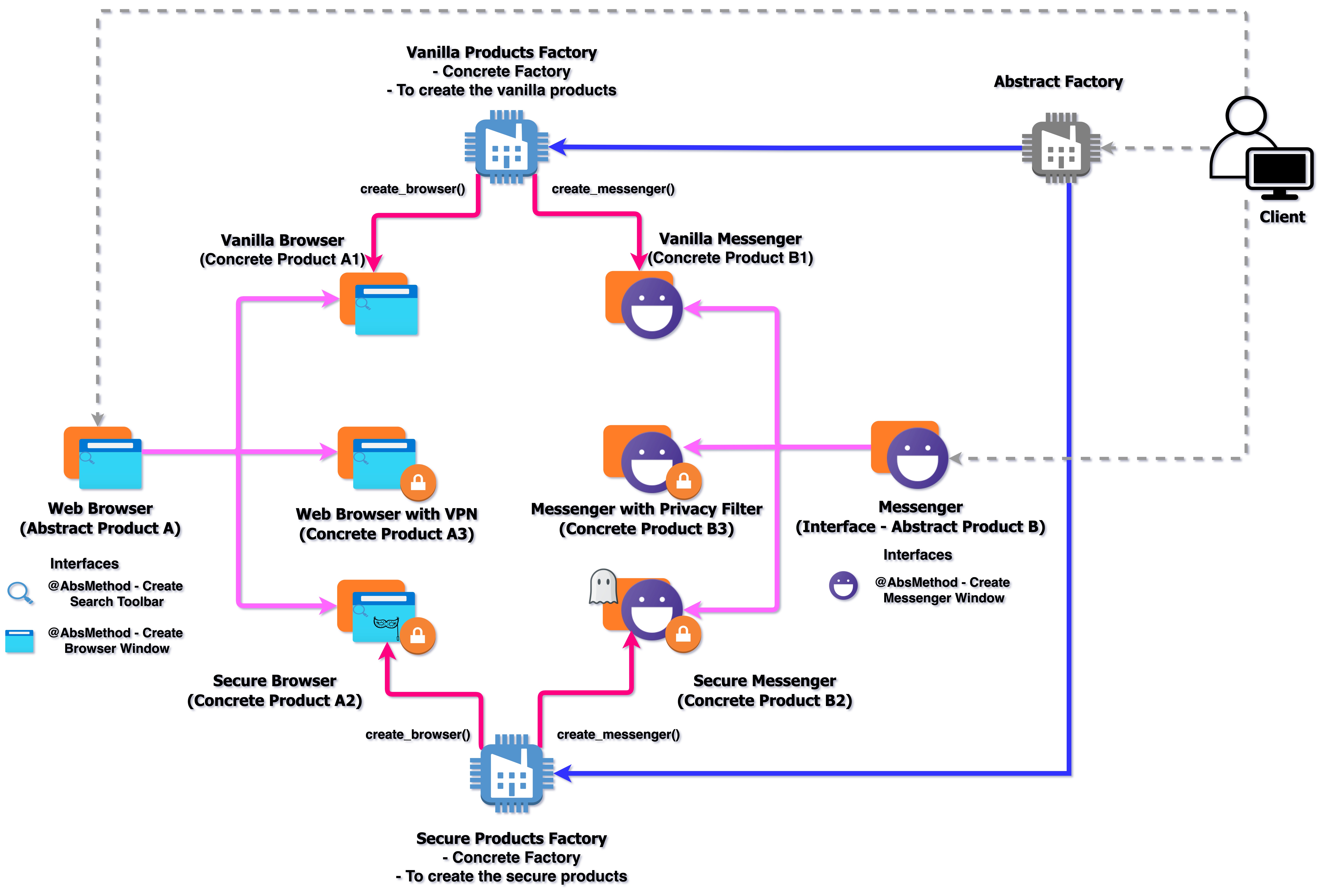
The Decorator pattern is useful when you want to extend the functionality of an object without changing its underlying implementation. The Adapter pattern allows objects with incompatible interfaces to work together by providing a compatible interface. It acts as a translator between two classes or objects that have incompatible interfaces. The Adapter pattern is useful when you want to integrate existing or legacy code into your application without modifying its source code. This planning is known as object-oriented design (OOD), and getting it right can be a challenge.
ABC works by decorating methods of the base class as abstract and then registering concrete classes as implementations of the abstract base. In fact, design patterns are simply ways to solve problems; they are independent of any programming language. You can implement most design patterns in a wide range of programming languages. A good solution is to specialize a general purpose implementation to provide an interface that is concrete to the application context. In this section, you will specialize ObjectFactory in the context of our music services, so the application code communicates the intent better and becomes more readable.
If we add more components, the interdependencies grow exponentially. If we change the underlying storage mechanism in the future, all code that directly accesses books will break. Each new action is a new command class, ensuring the system remains modular and easy to maintain. Builder can be valuable when creating complex Composite trees because its construction steps can be programmed to work recursively. In this implementation, we are using the Builder Pattern to create a form generator. Complex form structures, customization, validation rules, and styling can be systematically implemented using the Builder Pattern, simplifying the construction of intricate forms.
The example above exhibits all the problems you’ll find in complex logical code. Complex logical code uses if/elif/else structures to change the behavior of an application. Using if/elif/else conditional structures makes the code harder to read, harder to understand, and harder to maintain. This is a recurrent problem that makes Factory Method one of the most widely used design patterns, and it’s very important to understand it and know how apply it.
Creational patterns provides essential information regarding the Class instantiation or the object instantiation. Class Creational Pattern and the Object Creational pattern is the major categorization of the Creational Design Patterns. While class-creation patterns use inheritance effectively in the instantiation process, object-creation patterns use delegation effectively to get the job done. The pattern allows you to produce different types and representations of an object using the same construction code.
Wikipedia has a good catalog of design patterns with links to pages for the most common and useful patterns. The PandoraServiceBuilder implements the same interface, but it uses different parameters and processes to create and initialize the PandoraService. It also keeps the service instance around, so the authorization only happens once. Notice that SpotifyServiceBuilder keeps the service instance around and only creates a new one the first time the service is requested. This avoids going through the authorization process multiple times as specified in the requirements. The service returns an access code that should be used on any further communication.
No comments:
Post a Comment